Best Unity 3D Game Development Course in Kolkata
Are you ready to start your rewarding career as a proficient Unity game developer in Kolkata?
Join our professional Unity 3D game development course at Webskitters Academy for a lucrative kickstart into the IT domain.
Our course is well designed to meet the excellence of standards, industry demands, and skills to develop high-quality games. With our Unity 3D game development course in Kolkata, you will learn everything from tools and C# scripting to mastering the 3D game design and deployment process.
Rely on our expert-led course to gain hands-on experience and prepare for a dynamic career in game development.
Become the next proficient in C# programming language to build scripts.
Gain hands-on experience using Unity Editor in your game.
Master how to create 3D assets and textures for game environments and characters.
Implement realistic physics, including object collisions, gravity, and movements.
We help you learn Unity’s lighting system to enhance games’ visual appeal and atmosphere.
Learn how to animate characters, integrate motion capture data, and apply animations to game objects.
Learn advanced coding techniques, best practices for Unity scripts, and code optimisation strategies.
Develop multiplayer games with real-time online capabilities and network synchronisation.
Get ideas to optimise your games for smooth performance on different platforms.
Gain an understanding of creating intuitive, user-friendly interfaces.
Master to monetise your games through in-app purchases and ads to publish on various platforms.
Acquire knowledge to enhance Unity’s capabilities for developing immersive VR and AR experiences.
Gain an understanding of critical decision-making abilities to succeed professionally.
Master essential skills to collaborate with relevant professionals to bring your game projects to life.
Develop impactful skills to adapt to new technologies and game development trends as they emerge.
Grasp the art of explaining complex game development concepts to non-technical clients.
Learn how to work with diverse teams and interact with clients globally.
Prepare for interviews with mock sessions, guidance, and tips to impress potential employers.
The Technology You Will Learn in This Course
At Webskitters Academy, you will learn different tools and technologies to excel in the world of game development. These include Unity 3D, C#, Blender, Photon, Google VR & AR Tools, Visual Studio, Git & GitHub, Postman, and more.
Key Highlights of Our Unity 3D Game Development Course
Our Unity 3D Game Development Course in Kolkata is intended for people who find the game industry imaginative and engaging. Let us discover the key highlights of the course –
- Innovative and comprehensive syllabus that starts from the fundamentals to advanced techniques.
- Construction of 2D and 3D games, all from squares.
- Learn C# scripting in-game mechanics, physics, and AI.
- Develop a mobile game using touch inputs and accelerometer on an iPhone or iPad.
- Real-life version control with Git is used for managing game development projects.
- Design all player controllers, AI behaviours, and interactive game features.
- The physics system of Unity, scene management, and its user interface systems are all covered in the articles.
- Build a whole C# scripted game in Unity for Android with this.
Benefits of Our Unity 3D Game Development Course
By enrolling in Webskitters Academy’s Unity 3D Game Development course in Kolkata, you will gain the following benefits –
- Enhance your practical skills in game development to excel.
- Practical ideation and development of mobile games for use on the Android OS.
- It is beneficial for opening doors for a new job.
- Material can be accessed from anywhere, so studying is done at their leisure.
- Learning Git provides a strong basis for effective project management in both product and software design.
- Stay current and create modern projects by using the latest tools, technologies, techniques, and industry-standard methods.
- Build a strong portfolio with shining games to showcase your skills.
- Enhance your creativity and problem-solving skills by building dynamic, interactive gaming experiences.
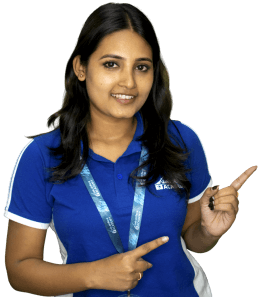
Download Course Module
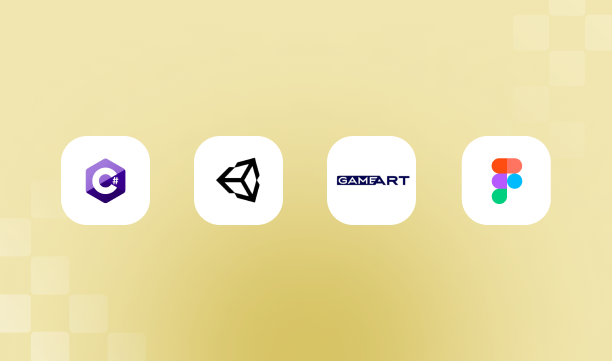
165,000
-
Minimum Eligibility:
B.Tech/M.Tech/BCA/MCA/Diploma /BSC CSE
-
Course Duration:
5 to 6 Months
-
Mode of Training:
Online/Offline
Get a Call Back
FAQ
1. How can I enrol in Kolkata's Unity 3D Game Development course?
To join our Unity 3D Game Development course at Webskitters Academy, you need to visit our website at https -//www.webskittersacademy.in/
Drop a query, and our team will contact you with further details to enrol in our course. You can also make direct contact via –
· Toll-Free Number – 18004199397
· https -//t.me/WAChatGroup
2. I am a beginner; can I join the Unity 3D Game Development course in Kolkata?
Yes! Our Unity 3D game development course in Kolkata at Webskitters Academy applies to every aspiring developer.
If you are a beginner and willing to master game development, this course is for you. We prefer a comprehensive learning approach, starting with the fundamentals and introducing advanced concepts, tools, and technologies.
We strive to build strong foundational skills and give maximum practical exposure.
3. Do you provide job placement assistance?
We ensure placement guidance for every candidate who takes a course at Webskitters Academy. Our expert team is dedicated to offering personalised grooming classes to prepare you for the job in top gaming companies.
4. Do you provide both online and offline training?
Yes! At Webskitters Academy, we offer online and offline classes in Kolkata. We believe in offering the best course with live classes. Our goal is to make individuals comfortable with flexible modes of learning. You can choose the mode according to your convenience and schedule.
5. Will I get practical experience during the course?
Yes! By joining our Unity 3D game development course in Kolkata, you will get practical exposure by working on live projects under expert mentorship. We prioritise a hands-on learning approach to give practical experience and to make you capable of handling real-world scenarios.